C/C++ 基礎知識
C 語言優秀學習網站
C 語言的注釋
- 單行注釋
/* comment goes here */
// comment goes here
- 多行注釋
/*
* comment goes here
*/
- Example
// 這是一個倒序 9x9 乘法表
#include <stdio.h>
int main()
{
/* 定義了 i,j,result 三個局部變量 */
int i, j, result;
for (i = 9; i > 0; i--) {
for (j = 1; j <= i; j++) {
printf("%d*%d=%d\t", i, j, i * j);
}
printf("\n");
}
return 0;
}
// 結果
/*
9*1=9 9*2=18 9*3=27 9*4=36 9*5=45 9*6=54 9*7=63 9*8=72 9*9=81
8*1=8 8*2=16 8*3=24 8*4=32 8*5=40 8*6=48 8*7=56 8*8=64
7*1=7 7*2=14 7*3=21 7*4=28 7*5=35 7*6=42 7*7=49
6*1=6 6*2=12 6*3=18 6*4=24 6*5=30 6*6=36
5*1=5 5*2=10 5*3=15 5*4=20 5*5=25
4*1=4 4*2=8 4*3=12 4*4=16
3*1=3 3*2=6 3*3=9
2*1=2 2*2=4
1*1=1
*/
C keywords
Keywords defined by ANSI and ISO C90.
auto double int struct
break else long switch
case enum register typedef
char extern return union
const float short unsigned
continue for signed void
default goto sizeof volatile
do if static while
Words added by ISO C99.(5 個)
_Bool _Imaginary restrict _Complex inline
Words added by ISO C11.(7 個)
Alignas _Generic _Thread_local _Alignof _Noreturn _Atomic _Static_assert
C 語言關鍵字
C 語言的標識符
C 語言規定,標識符可以是字母 (A~Z,a~z)
、數字 (0~9)
、下划線_
組成的字符串,並且第一個字符
必須是字母
或下划線
。
在使用標識符時還有注意以下幾點
-
(1) 標識符的長度最好不要超過 8 位,因為在某些版本的 C 中規定標識符前 8 位有效,當兩個標識符前 8 位相同時,則被認為是同一個標識符。
-
(2) 標識符是嚴格區分大小寫的。例如
Google
和google
是兩個不同的標識符。 -
(3) 標識符最好選擇有意義的英文單詞組成做到"見名知意",不要使用中文。
-
(4) 標識符不能是 C 語言的
關鍵字
。
C 語言數據類型
參考資料
在 C 編程語言中,數據類型分類如下...
- 主數據類型(基本數據類型或預定義數據類型)
- 派生數據類型(輔助數據類型或用戶定義的數據類型)
- 枚舉數據類型
- void 數據類型
Primary Datatypes
- Integer Datatype
- Floating Point Datatype
- Double Datatype
- Character Datatype
Integer Datatype
Floating Point Datatypes
- float
- double
Character Datatype
void Datatype
void 數據類型表示沒有值或沒有值。通常,void 用於指定不返回任何值的函數。我們還使用 void 數據類型來指定函數的空參數。
Enumerated Datatype
枚舉數據類型是用戶定義的數據類型,由整數常量組成,每個整數常量都有一個名稱。關鍵字enum
用於定義枚舉數據類型。
Derived Datatypes
- Arrays
- Structures
- Unions
- Enumeration
Output Functions in C
參考資料
C 編程語言提供以下內置輸出功能...
- 1.printf()
- 2.putchar()
- 3.puts()
- 4.fprintf()
printf() function
#include <stdio.h>
int main()
{
int i = 10;
float x = 5.5;
printf("%d %f\n", i, x);
return 0;
}
#include <stdio.h>
int main(){
int i = 10;
float x = 5.5;
printf("Integer value = %d, float value = %f\n",i, x);
return 0;
}
/* C 編程語言中的每個函數都必須具有返回值。printf()函數也有整數作為返回值。
printf()函數返回一個與其打印的字符總數相等的整數值。 */
#include <stdio.h>
int main(){
int i;
i = printf("btechsmartclass");
printf(" is %d number of characters.\n",i);
}
#include <stdio.h>
int main()
{
printf("Welcome to\n");
printf("btechsmartclass\n");
printf("the perfect website for learning\n");
return 0;
}
putchar() function
putchar()函數用於在輸出屏幕上顯示單個字符。putchar()函數打印作為參數傳遞給它的字符,並返回與返回值相同的字符。此功能僅用於打印單個字符。要打印多個字符,我們需要多次寫入或使用循環語句。
#include <stdio.h>
int main(){
char ch = 'A';
putchar(ch);
putchar('\n');
}
puts() function
puts()函數用於在輸出屏幕上顯示字符串。puts()函數打印字符串或字符序列,直到換行符。
#include <stdio.h>
int main(){
char name[30];
printf("\nEnter your favourite website: ");
gets(name);
puts(name);
}
C 語言中的常數
參考資料
在 C 編程語言中,常量類似於變量,但常量在程序執行期間僅保存一個值。這意味着,一旦將值賦值給常量,則在程序執行期間不能更改該值。將值分配給常量后,它將在整個程序中得到修復。常數可以定義如下...
常量是一個命名的內存位置,它在整個程序執行過程中只保存一個值。
In C programmig language, constant can be of any datatype like integer, floating point, character, string and double etc.
- 整數常量
例子:
125 -----> 十進制整數常量
O76 -----> 八進制整數常量
OX3A -----> 十六進制十進制整數常量
50u -----> 無符號整數常量
30l -----> 長整數常數
100ul -----> 無符號長整數常量
- 浮點常數
浮點常量必須包含整數和小數部分。有時它也可能包含指數部分。當浮點常量以指數形式表示時,該值必須以“e”或“E”為后綴。
例:浮點值 3.14 以指數形式表示為 3E-14。
- 字符常量
字符常量是用單引號括起來的符號。字符常量的最大長度為一個字符。
例:'A' '2' '+'
- 字符串常量
字符串常量是用雙引號括起來的字符,數字,特殊符號和轉義序列的集合。
我們在一行中定義字符串常量如下:“這是 btechsmartclass”
在 C 中創建常量
在 C 編程語言中,可以使用兩個概念創建常量...
- 1.使用 'const' 關鍵字
- 2.使用 '#define' 預處理器
1.使用 'const' 關鍵字
我們使用 'const' 關鍵字創建任何數據類型的常量。要創建常量,我們在變量聲明前加上 'const' 關鍵字。
使用 'const' 關鍵字創建常量的一般語法如下... const 數據類型 constantName; 要么 const 數據類型 constantName = value; 例:const int x = 10; 這里,'x' 是一個固定值為 10 的整數常數。
- 示例程序
#include <stdio.h>
void main(){
int i = 9 ;
const int x = 10 ;
i = 15 ;
x = 100 ; // creates an error
printf("i = %d\nx = %d", i, x ) ;
}
上面的程序給出了一個錯誤,因為我們試圖改變常量變量值(x = 100)。
2.使用 '#define' 預處理器
我們還可以使用 '#define' 預處理程序指令創建常量。當我們使用這個預處理器指令創建常量時,它必須在程序的開頭定義(因為所有預處理器指令必須在 gloabal 聲明之前寫入)。
我們使用以下語法使用 '#define' 預處理程序指令創建常量...
#define CONSTANTNAME value
例 #define PI 3.14
這里,PI 是一個值為 3.14 的常量
- 示例程序
#include <stdio.h>
#defien PI 3.14
void main(){
int r, area ;
printf("Please enter the radius of circle : ") ;
scanf("%d", &r) ;
area = PI * (r * r) ;
printf("Area of the circle = %d", area) ;
}
自增與自減運算符
自增運算符為“++”,其功能是使變量的值自增 1;
自減運算符為“--”,其功能是使變量值自減 1。
它們經常使用在循環中,自增自減運算符有以下幾種形式:
- Question 1
#include <stdio.h>
int main() {
int i = 3;
int a = i ++; // a = 3, i = 4
printf("a = %d, i = %d\n", a, i);
int b = ++ a; // b = 4, a = 4
printf("a = %d, b = %d\n", a, b);
return 0;
}
- Question 2
#include <stdio.h>
int main() {
int a = 10; // a 被賦值為 10
int b = ++ a; // b 值為 11,因為 a 是預先遞增的(現在 a = 11 且 b = 11)
int c = a ++; // c 值是 11,a 值是 12
printf("a = %d, b = %d, c = %d\n", a, b, c); // a = 12, b = 11, c = 11
return 0;
}
- Question 3
#include <stdio.h>
#include <conio.h>
int main() {
int x, a, b, c;
a = 2;
b = 4;
c = 5;
x = a -- + b ++ - ++ c ;
printf("x = %d\n", x); // x = 0
printf("a = %d,b = %d,c = %d\n", a, b, c); // a = 1,b = 5,c = 6
getch();
return 0;
}
- Question 4
#include <stdio.h>
#include <conio.h>
int main() {
int a, b, c, d;
a = 2, c = 2;
b = a++*++a;
d = ++c * c++;
printf("a = %d, b = %d\n", a, b);
printf("c = %d, d = %d\n", c, d);
getch();
return 0;
}
/*
gcc 5 result
a = 4, b = 9
c = 4, d = 9
*/
- Question 5
/*
添加 3 個變量可以分解為 2 個步驟。
temp = ++ a + ++ a;
b = temp + ++ a;
第一個++ a 使值為 6,第二個++ a 使它成為 7。因為 temp 中使用的兩個變量都指向 a,temp = 7 + 7 = 14。
下一步很簡單,b = temp + 8,這使得 b = 22。
*/
#include <stdio.h>
#include <conio.h>
int main() {
int a = 5, b;
b = ++a + ++a + ++a;
printf("a = %d,b = %d", a, b); // a = 8,b = 22
getch();
return 0;
}
- Question 6
面試官最喜歡的技術面試問題之一:
int a = 1;
int b = 2;
int c == a +++ b;
它編譯嗎?如果沒有,需要改變什么。運行后 a,b,c 的值為多少?
#include <stdio.h>
#include <conio.h>
int main() {
int a = 1;
int b = 2;
int c = a +++ b;
printf("a = %d,b = %d,c = %d\n", a, b, c); // a = 2,b = 2,c = 3
getch();
return 0;
}
注意:無論是 a++還是++a 都等同於 a=a+1,在表達式執行完畢后 a 的值都自增了 1,無論是 a--還是--a 都等同於 a=a-1,在表達式執行完畢后 a 的值都自減少 1。
C 語言運算符優先級
運算符優先級確定表達式的執行順序,並決定如何計算表達式。某些運算符的優先級高於其他運算符; 例如,乘法運算符的優先級高於加法運算符。 例如,x = 7 + 3 * 2; 這里,x 被賦值為 13,而不是 20,因為 operator * 的優先級高於 +,所以它首先乘以 3 * 2 然后加到 7 中。 此處,具有最高優先級的運算符顯示在表的頂部,具有最低優先級的運算符顯示在底部。在表達式中,將首先執行更高優先級的運算符。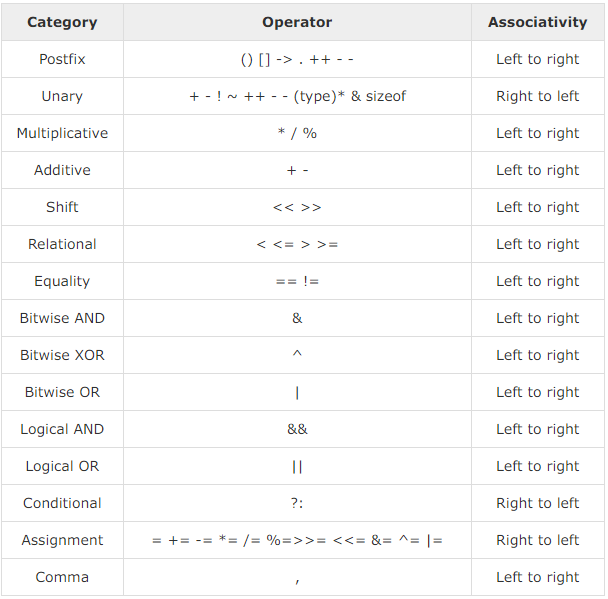